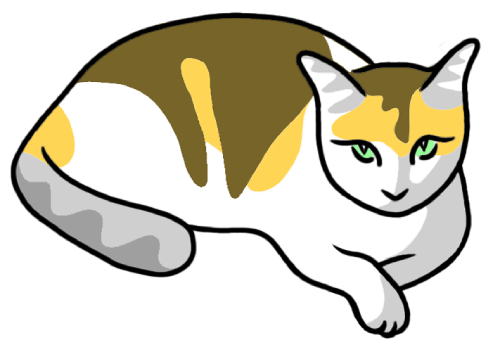
PHP Functions via Schema
PHP Functions via Schema
This extension adds fields and directives to the GraphQL schema which expose functionalities commonly found in programming languages (such as PHP).
This query, containing a variety of function fields and directives:
{
_intAdd(add: 15, to: 56)
_intArraySum(array: [1, 2, 3, 4, 5])
_arrayJoin(array: ["Hello", "to", "everyone"], separator: " ")
_arrayItem(array: ["one", "two", "three", "four", "five"], position: 3)
_arraySearch(array: ["uno", "dos", "tres", "cuatro", "cinco"], element: "tres")
_arrayUnique(array: ["uno", "dos", "uno", "tres", "cuatro", "dos", "cinco", "dos"])
_arrayMerge(arrays: [["uno", "dos", "uno"], ["tres", "cuatro", "dos", "cinco", "dos"]])
_arrayDiff(arrays: [["uno", "dos"], ["tres", "cuatro", "dos"]])
_arrayAddItem(array: ["uno", "dos"], value: "tres")
_arraySetItem(array: ["uno", "dos"], index: 0, value: "tres")
_arrayKeys(array: ["uno", "dos", "tres"])
_arrayLength(array: ["uno", "dos", "tres"])
_strReplace(search: "https://", replaceWith: "http://", in: "https://gatographql.com")
_strReplaceMultiple(search: ["https://", "gato"], replaceWith: ["http://", "dog"], in: "https://gatographql.com")
_strRegexReplace(searchRegex: "/^https?:\\/\\//", replaceWith: "", in: "https://gatographql.com")
_strRegexReplaceMultiple(searchRegex: ["/^https?:\\/\\//", "/([a-z]*)/"], replaceWith: ["", "$1$1"], in: "https://gatographql.com")
_strStartsWith(search: "orld", in: "Hello world")
_strEndsWith(search: "orld", in: "Hello world")
_strUpperCase(text: "Hello world")
_strLowerCase(text: "Hello world")
_strTitleCase(text: "Hello world")
falseToTrue: _echo(value: false) @boolOpposite
trueToFalse: _echo(value: true) @boolOpposite
plusOne: _echo(value: 2) @intAdd(number: 1)
objectAddEntry: _echo(value: {
user: "Leo",
contact: {
email: "leo@test.com"
}
})
@objectAddEntry(key: "phone", value: "+0929094229", underPath: "contact")
@objectAddEntry(key: "methods", value: {}, underPath: "contact")
@objectAddEntry(key: "card", value: true, underPath: "contact.methods")
upperCase: _echo(value: "Hello world") @strUpperCase
lowerCase: _echo(value: "Hello world") @strLowerCase
titleCase: _echo(value: "Hello world") @strTitleCase
append: _echo(value: "Hello world") @strAppend(string: "!!!")
prepend: _echo(value: "Hello world") @strPrepend(string: "!!!")
arraySplice: _echo(value: ["uno", "dos", "tres"]) @arraySplice(offset: 1)
arraySpliceWithLength: _echo(value: ["uno", "dos", "tres"]) @arraySplice(offset: 1, length: 1)
arraySpliceWithReplacement: _echo(value: ["uno", "dos", "tres"]) @arraySplice(offset: 1, replacement: ["cuatro", "cinco"])
arraySpliceWithLengthAndReplacement: _echo(value: ["uno", "dos", "tres"]) @arraySplice(offset: 1, length: 1, replacement: ["cuatro", "cinco"])
arrayUnique: _echo(value: ["uno", "dos", "uno", "tres", "cuatro", "dos", "cinco", "dos"]) @arrayUnique
arrayMerge: _echo(value: ["uno", "dos", "uno"]) @arrayMerge(with: ["tres", "cuatro", "dos", "cinco", "dos"])
arrayDiff: _echo(value: ["uno", "dos"]) @arrayDiff (against: ["tres", "cuatro", "dos"])
arrayFilter: _echo(value: ["uno", "dos", null, "tres", "", "dos", []]) @arrayFilter
objectKeepProperties: _echo(value: { user: "Leo", email: "leo@test.com" } )
@objectKeepProperties(
keys: ["user"]
)
}
...produces:
{
"data": {
"_intAdd": 71,
"_intArraySum": 15,
"_arrayJoin": "Hello to everyone",
"_arrayItem": "four",
"_arraySearch": 2,
"_arrayUnique": [
"uno",
"dos",
"tres",
"cuatro",
"cinco"
],
"_arrayMerge": [
"uno",
"dos",
"uno",
"tres",
"cuatro",
"dos",
"cinco",
"dos"
],
"_arrayDiff": [
"uno"
],
"_arrayAddItem": [
"uno",
"dos",
"tres"
],
"_arraySetItem": [
"tres",
"dos"
],
"_arrayKeys": [
0,
1,
2
],
"_arrayLength": 3,
"_strReplace": "http://gatographql.com",
"_strReplaceMultiple": "http://doggraphql.com",
"_strRegexReplace": "gatographql.com",
"_strRegexReplaceMultiple": "gatographqlgatographql.comcom",
"_strStartsWith": false,
"_strEndsWith": true,
"_strUpperCase": "HELLO WORLD",
"_strLowerCase": "hello world",
"_strTitleCase": "Hello World",
"falseToTrue": true,
"trueToFalse": false,
"plusOne": 3,
"objectAddEntry": {
"user": "Leo",
"contact": {
"email": "leo@test.com",
"phone": "+0929094229",
"methods": {
"card": true
}
}
},
"upperCase": "HELLO WORLD",
"lowerCase": "hello world",
"titleCase": "Hello World",
"append": "Hello world!!!",
"prepend": "!!!Hello world",
"arraySplice": [
"uno"
],
"arraySpliceWithLength": [
"uno",
"tres"
],
"arraySpliceWithReplacement": [
"uno",
"cuatro",
"cinco"
],
"arraySpliceWithLengthAndReplacement": [
"uno",
"cuatro",
"cinco",
"tres"
],
"arrayUnique": [
"uno",
"dos",
"tres",
"cuatro",
"cinco"
],
"arrayMerge": [
"uno",
"dos",
"uno",
"tres",
"cuatro",
"dos",
"cinco",
"dos"
],
"arrayDiff": [
"uno"
],
"arrayFilter": [
"uno",
"dos",
"tres",
"dos"
],
"objectKeepProperties": {
"user": "Leo"
}
}
}