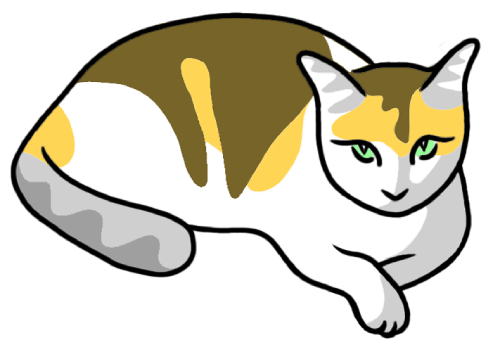
HTTP Client
HTTP Client
Addition of fields to the GraphQL schema to execute HTTP requests against a webserver and fetch their response:
_sendJSONObjectItemHTTPRequest
_sendJSONObjectItemHTTPRequests
_sendJSONObjectCollectionHTTPRequest
_sendJSONObjectCollectionHTTPRequests
_sendHTTPRequest
_sendHTTPRequests
_sendGraphQLHTTPRequest
_sendGraphQLHTTPRequests
It supports fetching any piece of data from the HTTP response:
{
_sendHTTPRequest(
input: {
url: "https://mysite.com/wp-json/wp/v2/comments/11/?_fields=id,date,content"
}
) {
statusCode
contentType
headers
body
contentLengthHeader: header(name: "Content-Length")
cacheControlHeader: header(name: "Cache-Control")
}
}
...producing:
{
"data": {
"_sendHTTPRequest": {
"statusCode": 200,
"contentType": "application\/json; charset=UTF-8",
"headers": {
"Access-Control-Allow-Headers": "Authorization, X-WP-Nonce, Content-Disposition, Content-MD5, Content-Type",
"Access-Control-Expose-Headers": "X-WP-Total, X-WP-TotalPages, Link",
"Allow": "GET",
"Cache-Control": "max-age=300,no-store",
"Content-Length": "508"
},
"body": "{\"id\":11,\"date\":\"2020-12-12T04:09:36\",\"content\":{\"rendered\":\"<p>Wow, this sounds awesome!<\\\/p>\\n\"},\"_links\":{\"self\":[{\"href\":\"https:\\\/\\\/mysite.com\\\/wp-json\\\/wp\\\/v2\\\/comments\\\/11\"}],\"collection\":[{\"href\":\"https:\\\/\\\/mysite.com\\\/wp-json\\\/wp\\\/v2\\\/comments\"}],\"author\":[{\"embeddable\":true,\"href\":\"https:\\\/\\\/mysite.com\\\/wp-json\\\/wp\\\/v2\\\/users\\\/3\"}],\"up\":[{\"embeddable\":true,\"post_type\":\"post\",\"href\":\"https:\\\/\\\/mysite.com\\\/wp-json\\\/wp\\\/v2\\\/posts\\\/28\"}]}}",
"contentLengthHeader": "508",
"cacheControlHeader": "max-age=300,no-store"
}
}
}
It supports connecting to a REST endpoint, converting the response to JSON:
{
_sendJSONObjectItemHTTPRequest(
input: {
url: "https://mysite.com/wp-json/wp/v2/posts/?per_page=3&_fields=id,type,title,date"
}
)
}
...producing:
{
"data": {
"_sendJSONObjectItemHTTPRequest": [
{
"id": 1692,
"date": "2022-04-26T10:10:08",
"type": "post",
"title": {
"rendered": "My Blogroll"
}
},
{
"id": 1657,
"date": "2020-12-21T08:24:18",
"type": "post",
"title": {
"rendered": "A tale of two cities – teaser"
}
},
{
"id": 1499,
"date": "2019-08-08T02:49:36",
"type": "post",
"title": {
"rendered": "COPE with WordPress: Post demo containing plenty of blocks"
}
}
]
}
}
It supports connecting to a GraphQL endpoint, converting the response to JSON:
{
_sendGraphQLHTTPRequest(
input: {
endpoint: "https://mysite.com/api/graphql/"
query: """
query GetPosts($postIDs: [ID]!) {
posts(filter: { ids: $postIDs }) {
id
title
}
}
"""
variables: [
{
name: "postIDs",
value: [1, 1499]
}
]
}
)
}
...producing:
{
"data": {
"_sendGraphQLHTTPRequest": {
"data": {
"posts": [
{
"id": 1499,
"title": "COPE with WordPress: Post demo containing plenty of blocks"
},
{
"id": 1,
"title": "Hello world!"
}
]
}
}
}
}