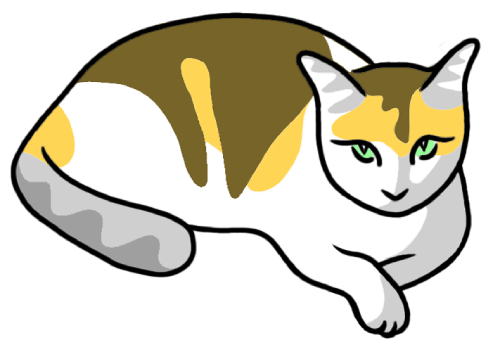
Field Value Iteration and Manipulation
Field Value Iteration and Manipulation
Addition of meta directives to the GraphQL schema, for iterating and manipulating the value elements of array and object fields:
@underArrayItem
@underJSONObjectProperty
@underEachArrayItem
@underEachJSONObjectProperty
@objectClone
@underArrayItem
makes the nested directive be applied on a specific item from the array.
query {
posts {
categoryNames
@underArrayItem(index: 0)
@strUpperCase
}
}
...produces:
{
"data": {
"posts": {
"categoryNames": [
"NEWS",
"sports"
]
}
}
}
@underJSONObjectProperty
makes the nested directive receive an entry from the queried JSON object.
query {
postData: _sendJSONObjectItemHTTPRequest(
url: "https://newapi.getpop.org/wp-json/wp/v2/posts/1/?_fields=id,type,title,date"
)
@underJSONObjectProperty(by: { path: "title.rendered" })
@strUpperCase
}
...produces:
{
"data": {
"postData": {
"id": 1,
"date": "2019-08-02T07:53:57",
"type": "post",
"title": {
"rendered": "HELLO WORLD!"
}
}
}
}
@underEachArrayItem
iterates over the array items from some field in the queried entity, and executes the nested directive(s) on each of them.
query {
posts {
id
title
categoryNames
frenchCategoryNames: categoryNames
@underEachArrayItem
@strTranslate(
from: "en",
to: "fr"
)
}
}
...produces:
{
"data": {
"posts": [
{
"id": 662,
"title": "Explaining the privacy policy",
"categoryNames": [
"Not categorized"
],
"frenchCategoryNames": [
"Non classé"
]
},
{
"id": 28,
"title": "HTTP caching improves performance",
"categoryNames": [
"Advanced"
],
"frenchCategoryNames": [
"Avancé"
]
},
{
"id": 25,
"title": "Public or Private API mode, for extra security",
"categoryNames": [
"Resource",
"Blog",
"Advanced"
],
"frenchCategoryNames": [
"Ressource",
"Blog",
"Avancé"
]
}
]
}
}
@underEachJSONObjectProperty
is similar to @underEachArrayItem
, but operating on JSONObject
elements.
{
_echo(
value: {
first: "hello",
second: "world",
third: null
}
)
@underEachJSONObjectProperty
@default(value: "")
}
...produces:
{
"data": {
"_echo": {
"first": "hello",
"second": "world",
"third": ""
}
}
}